- As an Open Source Developer working at Red Hat, I strive to share my knowledge on computer science, mathematics, and technology through articles. If you’d like to stay in the loop with the latest content from this blog, I invite you to subscribe to my RSS feed.
- For a glimpse into my journey and the projects I’ve been involved in, feel free to check my resume.
brag: A Command Line Tool for Building a Bragging Document
Inspired by Julia Evan’s blog Get your work recognized: write a brag document I created this tool to keep a record of my brags in Markdown Format and refer them later to create a formatted bragging document. A bragging or hype document is created to keep a record of achievements over time. This document/information extracted from this document could be shared with managers or used for self-reflection and improvement. I refer to it during my daily standups and weekly sync-up meetings....
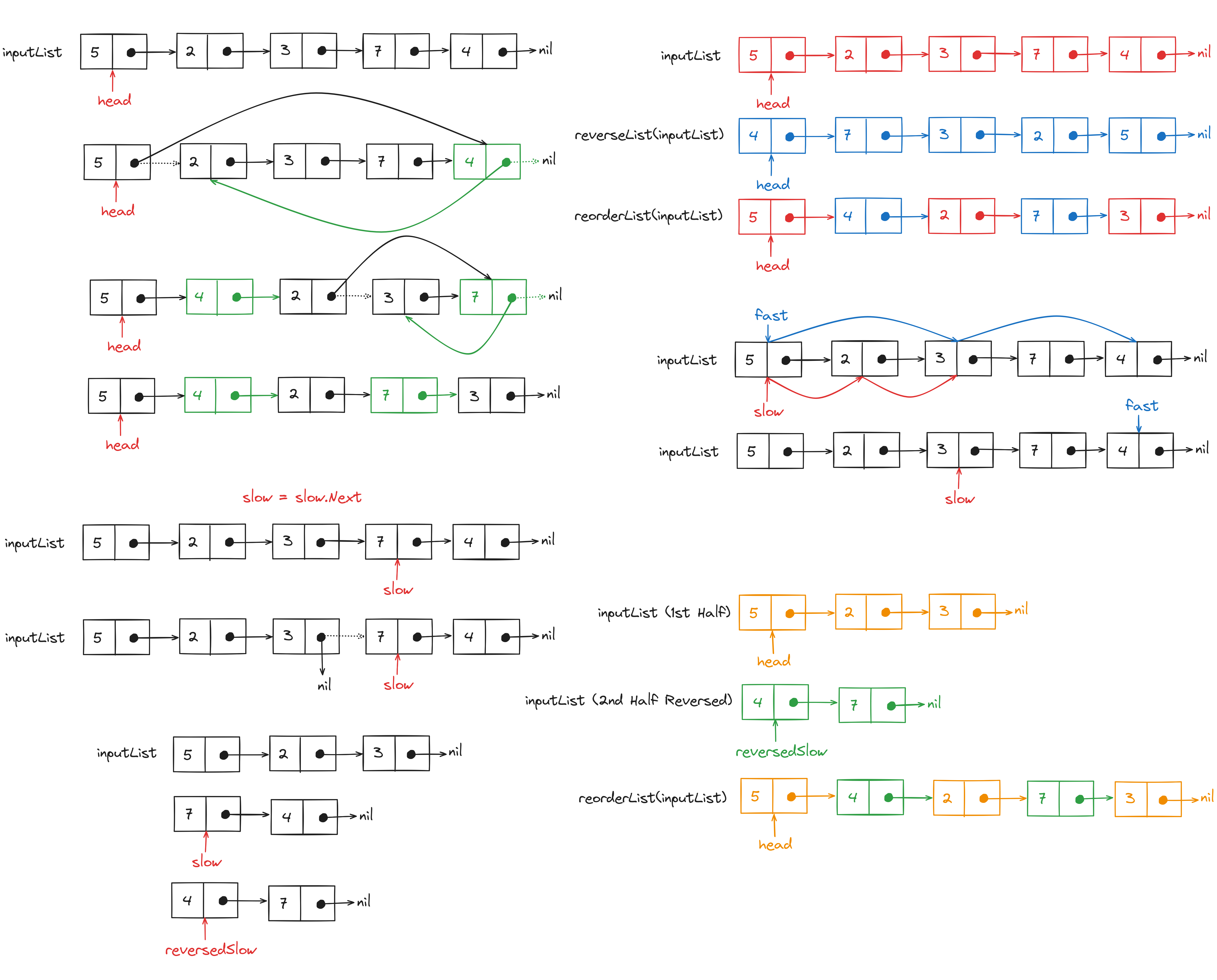
Reorder Linked Lists
Problem Statement We have to implement the reorderList function that takes the head node of a linked list as input and reorders its nodes in the format specified below. Given an input list like the following: $$Node_0 \rightarrow Node_1 \rightarrow Node_2 \rightarrow \dots \rightarrow Node_{(n-2)} \rightarrow Node_{(n-1)} \rightarrow Node_n$$ the reorderList function should reorder its nodes as: $$Node_0 \rightarrow Node_n \rightarrow Node_1 \rightarrow Node_{(n-1)} \rightarrow Node_2 \rightarrow Node_{(n-2)} \rightarrow \dots$$...
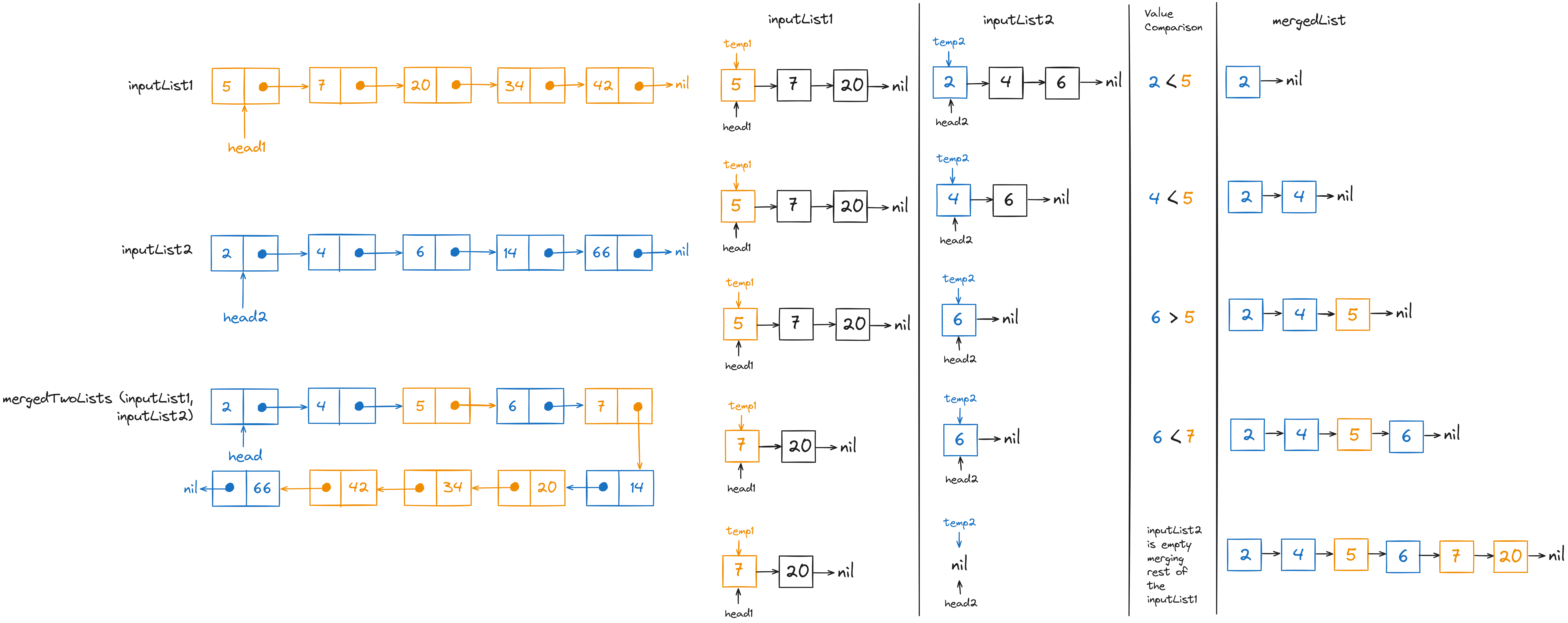
Merge Two Sorted Linked Lists
Problem Statement We have to implement the mergeTwoLists function that takes the head nodes of two sorted linked lists as input and returns the head node of the merged linked list as the output. Optimal Solution To merge both linked lists we can use the two-pointer approach by maintaining an iterator on both linked lists and comparing their values. The smaller value will be selected and inserted at the end of a new list....
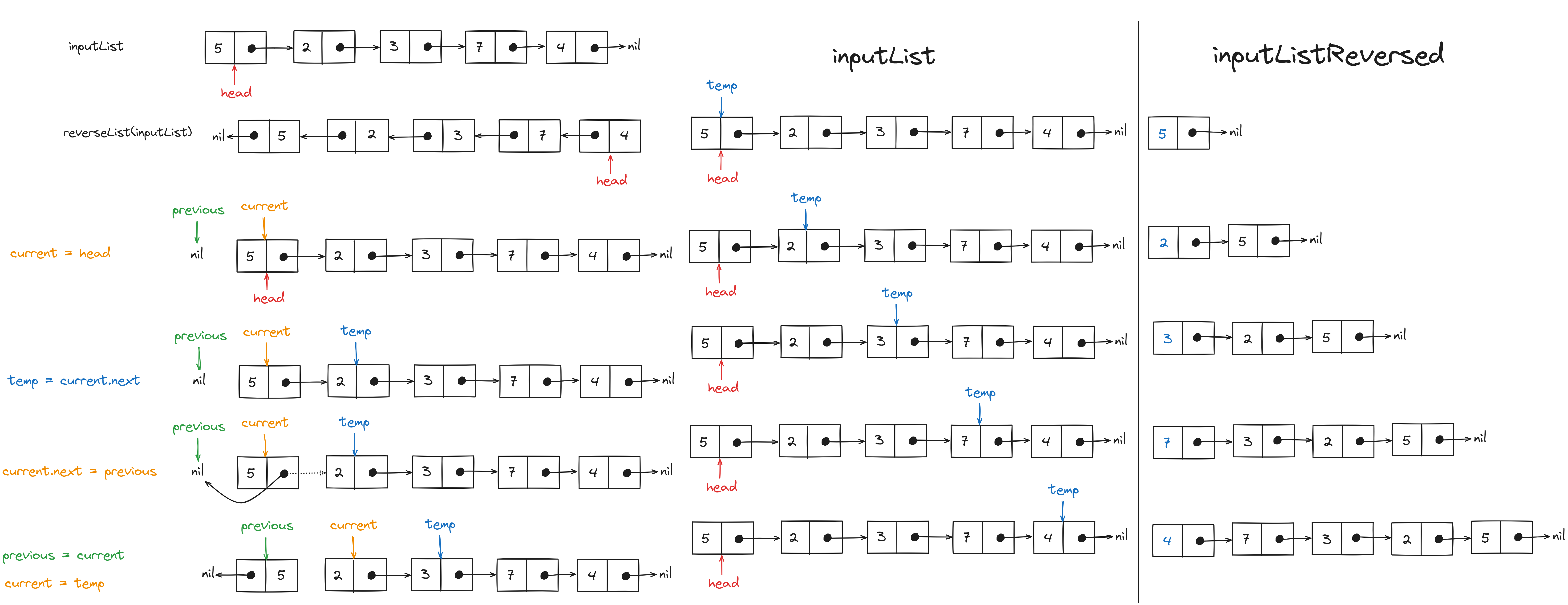
Reversing Linked Lists
Problem Statement We have to implement the reverseList function that takes the head node of a linked list as an input and returns the head node of the reversed linked list in the output. Brute Force Solution If we iterate over the input linked list and insert its value at the beginning of a new list the result would be a reversed linked list. Psuedo-code for the Brute Force Solution reversedLinkedList = LinkedList() temp = linked_list....
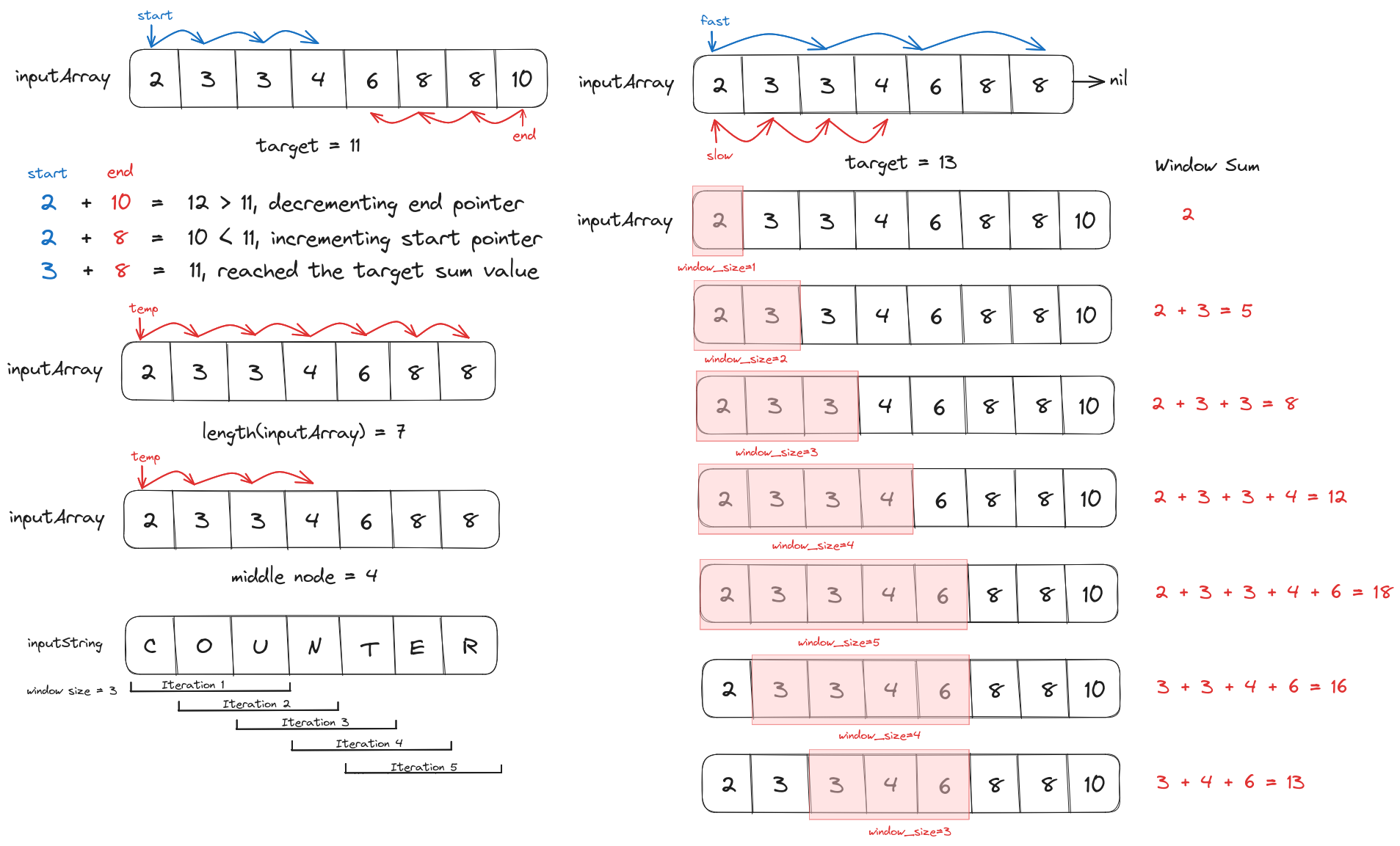
Two-Pointer Approach
To solve problems like Two Sums, Product Except Self, or Contains Duplicate we have to access multiple values at the same time from a sequential data structure (for example, a Linked List or an Array). The initial instinct while solving these problems is to use nested loops where each layer of the loop will maintain a different iterator on the data structure. But this approach does not scale well with the size of input as a single order of nested loops has $O(n^2)$ worst-case time complexity....